3 回答
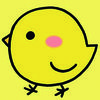
TA贡献1906条经验 获得超10个赞
(要了解更多 google 引号中的任何内容 :)
使用“while 循环”创建“游戏循环”。我从用 python 制作小游戏中学到了这个技巧。此外,您想学习使用“类”作为逻辑,并且可以使用“OOP 概念”改进代码。T 代码已经过测试并且可以工作。
import random
#Create a "function" that meets the requirements of a "game loop"
def gameloop():
game = input("Want to play Rock Paper Scissors? (Y/N) ")
if game == "Y":
#Create a "while loop" to host the logic of the game.
#Each If statement will enable one "rule" of the game logic.
#game logic could be redesigned as an "Event".
#You can add a game "Event System" to your future project backlog
winner = False
while not winner:
print("1 = Rock, 2 = Paper, 3 = Scissors")
print('')
user = int(
input("You have one chance of beating me. Input a number. "))
print('')
computer = random.randint(1, 3)
if user == 1 and computer == 2:
print("You lose! You played Rock and I played Paper!")
winner = True
elif user == 1 and computer == 3:
print("You win! You played Rock and I played Scissors!")
winner = True
elif user == 2 and computer == 1:
print("You win! You played Paper and I played Rock!")
winner = True
elif user == 2 and computer == 3:
print("You lose! You played Paper and I played Scissors!")
winner = True
elif user == 3 and computer == 1:
print("You lose! You played Scissors and I played Rock!")
winner = True
elif user == 3 and computer == 2:
print("You win! You played Scissors and I played Paper!")
winner = True
elif user == 1 and computer == 1:
print("Must play again! We both played Rock!")
elif user == 2 and computer == 2:
print("Must play again! We both played Paper!")
elif user == 3 and computer == 3:
print("Must play again! We both played Scissors!")
else:
print("Not a number.")
else:
print("game....over?")
gameloop()
我也花时间做了一个 OOP 类示例!它可以通过多种方式进行优化。但是,我希望它能向您展示下一个学习水平!我也希望它能帮助您了解所有您可以在学习时应用的疯狂设计模式和游戏编程技术。
import random
# we can create a player class to be used as an "interface" as we design the games logic
# this will let us scale the features be build in our game
# in this case i will leave it to you to add Wins to the scoreboard to help you learn
class player:
# your games requerment wants your players to make a choice from 1-3
choice = 0
# your games may requerment a player to be defined as the winner
win = 0
# your games may requerment a player to be defined as the losser
loss = 0
class game:
# by using classes and OOP we can scale the data and logic of your game
# here we create instances of the class player and define new objects based on your "requerments"
# your "requerments" where to have one Computer as a player, and one user as a player
computer = player()
user = player()
# this "function" will create a Scoreboard feature that can be called in the 'game loop' or in a future "event" of the game.
# Like a "Game Stats stage" at the end of the game
def Scoreboard(self, computer, user):
Computer = computer.loss
User = user.loss
print("+============= FINAL RESULTS: SCOREBOARD!! ======+ ")
print(" ")
print("Computer losses: ", Computer)
print("Player losses: ", User)
print(" ")
# Create a "function" that meets the requirements of a "game loop"
def main_loop(self, computer, user):
gameinput = input("Want to play Rock Paper Scissors? (Y/N) ")
if gameinput == "Y":
# Create a "while loop" to host the logic of the game.
# Each If statement will enable one "rule" of the game logic.
# game logic could be redesigned as an "Event".
# You can add a game "Event System" to your future project backlog
winner = False
while not winner:
print("1 = Rock, 2 = Paper, 3 = Scissors")
print('')
# we create 'Player1' as the user
Player1 = user
# we change the 'Player1' 'choice' to the user input
Player1.choice = int(
input("You have one chance of beating me. Input a number. "))
print('')
# we pull in to the game the computer player and call them 'Player1'
Player2 = computer
# we change the 'Player2' 'choice' to a random number
Player2.choice = random.randint(1, 3)
if user.choice == 1 and computer.choice == 2:
print("You lose! You played Rock and I played Paper!")
winner = True
user.loss += 1
elif user.choice == 1 and computer.choice == 3:
print("You win! You played Rock and I played Scissors!")
winner = True
computer.loss += 1
elif user.choice == 2 and computer.choice == 1:
print("You win! You played Paper and I played Rock!")
winner = True
computer.loss += 1
elif user.choice == 2 and computer.choice == 3:
print("You lose! You played Paper and I played Scissors!")
winner = True
user.loss += 1
elif user.choice == 3 and computer.choice == 1:
print("You lose! You played Scissors and I played Rock!")
winner = True
user.loss += 1
elif user.choice == 3 and computer.choice == 2:
print("You win! You played Scissors and I played Paper!")
winner = True
computer.loss += 1
elif user.choice == 1 and computer.choice == 1:
print("Must play again! We both played Rock!")
elif user.choice == 2 and computer.choice == 2:
print("Must play again! We both played Paper!")
elif user.choice == 3 and computer.choice == 3:
print("Must play again! We both played Scissors!")
else:
print("Not a number.")
# by returning "self" you call the same 'instances' of game that you will define below
return self.Scoreboard(user, computer)
else:
print("game....over?")
# define Instance of game as "test_game"
test_game = game()
# run game loop
test_game.main_loop()
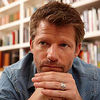
TA贡献1856条经验 获得超17个赞
一种方法是使用 while 循环,在满足条件时中断。
while True:
if (condition):
print("")
break
...
while 语句重复循环,直到满足其中一个条件。break 语句使程序退出循环并继续执行下一个可执行语句。
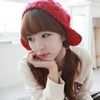
TA贡献1876条经验 获得超7个赞
您可以将整个 if-elif-block 放在一个 while 循环中,该循环将重复直到您有一个赢家。为了确定是否有赢家,使用布尔变量。
winner = False
while not winner:
if ...... ## Your if-elif-block
elif user == 1 and computer == 2:
print("You lose! You played Rock and I played Paper!")
winner = True
## Your remaining if-elif-block
您只需将命令放在winner=True具有获胜者条件的命令块中。因此,循环将继续,直到您达到这些条件之一。
您还可以选择使用更高级的获胜者变量(0 表示平局,1 表示玩家,2 表示计算机)来使用再见消息中的值。
添加回答
举报