3 回答
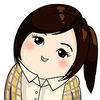
TA贡献1836条经验 获得超3个赞
编写代码时,始终尝试遵循 KISS 原则。保持简单愚蠢。您迷失在嵌套的 for-if while 循环中,很难找出哪里出了问题。
另一个原则是:不要让多个任务使您的方法超载。使用小而简单的方法,一次只执行一项任务。例如,下面我已将reversePhrase和reverseWord放入自己的方法中。这可以帮助您创建一个干净的 main 方法。
public static void main(String args[]) {
Scanner userInput = new Scanner(System.in);
System.out.print("Enter a word or phrase: ");
String str = userInput.nextLine();
//if input contains spaces call reversePhrase otherwise reverseWord
//str.contains(" ") is IMO cleaner, but you can change it to str.indexOf(" ") > -1 if you find it better
if(str.contains(" ")){
System.out.println(reversePhrase(str));
}
else{
System.out.println(reverseWord(str));
}
}
private static String reverseWord(String input) {
String result = "";
for(int i = input.length()-1; i >= 0; i--){
result = result + input.charAt(i);
}
return result;
}
private static String reversePhrase(String input) {
String result = "";
while(input.contains(" ")){
result = result + input.substring(input.lastIndexOf(" ")+1) + " ";
input = input.substring(0, input.lastIndexOf(" "));
}
return result + input;
}
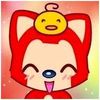
TA贡献1864条经验 获得超6个赞
在你的 while 循环中:
while(position >= 0){ //wasn't sure what to put for this parameter
reverseLetter = str.charAt(position); // position stays the same
reverseWord = reverseLetter + reverseWord;
}
它不会改变 的值position。(position永远不会是 0)我建议position--在最后添加,如下所示:
while(position >= 0){ //wasn't sure what to put for this parameter
reverseLetter = str.charAt(position); // position stays the same
reverseWord = reverseLetter + reverseWord;
position--;
}
它会改变变量的值position。
另外,您的代码中有一if和一。else if我建议更改else if为,else因为这样做毫无意义:
boolean randomBoolean = new java.util.Random().nextBoolean();
if(randomBoolean){...}
else if(!randomBoolean){...} // If randomBoolean == false, then this code will execute anyway
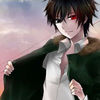
TA贡献1895条经验 获得超7个赞
我已经删除了其中一个for循环,因为您不需要它。另外,while针对一个单词的情况进行循环。对于第一种情况,您可以使用另一个字符串来临时保存最后一个单词。结果如下:
public static void main(String[] args) {
Scanner userInput = new Scanner(System.in);
System.out.print("Enter a word or phrase: ");
String str = userInput.nextLine(); //user-input string
String reversePhrase = ""; //placeholder for reversed phrase
String reverseWord = ""; //placeholder for reversed word
char reverseLetter; //placeholder for letters in reversed word
final String space = " ";
if(str.contains(space)) {
while(str.contains(space))
{
int i = str.lastIndexOf(space);
String lastWord = str.substring(i);
str = str.substring(0, i);
reversePhrase += lastWord;
}
//We add the first word
reversePhrase = reversePhrase + space + str;
System.out.println(reversePhrase.trim());
}
else {
for(int position = str.length() - 1; position >= 0; position --) {
reverseLetter = str.charAt(position);
reverseWord = reverseWord + reverseLetter;
}
System.out.println(reverseWord);
}
}
添加回答
举报