获取通用字典指定值的多个键?很容易从.NET通用字典中获得键的值:Dictionary<int, string> greek = new Dictionary<int, string>();greek.Add(1, "Alpha");greek.Add(2, "Beta");string secondGreek = greek[2]; // Beta但是,尝试获取给定值的键并不是那么简单,因为可能有多个键:int[] betaKeys = greek.WhatDoIPutHere("Beta"); // expecting single 2
3 回答
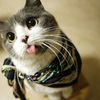
慕仙森
TA贡献1827条经验 获得超7个赞
using System;using System.Collections.Generic;using System.Text;class BiDictionary<TFirst, TSecond>{ IDictionary<TFirst, IList<TSecond>> firstToSecond = new Dictionary<TFirst, IList<TSecond>>(); IDictionary<TSecond, IList<TFirst>> secondToFirst = new Dictionary<TSecond, IList<TFirst>>(); private static IList<TFirst> EmptyFirstList = new TFirst[0]; private static IList<TSecond> EmptySecondList = new TSecond[0]; public void Add(TFirst first, TSecond second) { IList<TFirst> firsts; IList<TSecond> seconds; if (!firstToSecond.TryGetValue(first, out seconds)) { seconds = new List<TSecond>(); firstToSecond[first] = seconds; } if (!secondToFirst.TryGetValue(second, out firsts)) { firsts = new List<TFirst>(); secondToFirst[second] = firsts; } seconds.Add(second); firsts.Add(first); } // Note potential ambiguity using indexers (e.g. mapping from int to int) // Hence the methods as well... public IList<TSecond> this[TFirst first] { get { return GetByFirst(first); } } public IList<TFirst> this[TSecond second] { get { return GetBySecond(second); } } public IList<TSecond> GetByFirst(TFirst first) { IList<TSecond> list; if (!firstToSecond.TryGetValue(first, out list)) { return EmptySecondList; } return new List<TSecond>(list); // Create a copy for sanity } public IList<TFirst> GetBySecond(TSecond second) { IList<TFirst> list; if (!secondToFirst.TryGetValue(second, out list)) { return EmptyFirstList; } return new List<TFirst>(list); // Create a copy for sanity }}class Test{ static void Main() { BiDictionary<int, string> greek = new BiDictionary<int, string>(); greek.Add(1, "Alpha"); greek.Add(2, "Beta"); greek.Add(5, "Beta"); ShowEntries(greek, "Alpha"); ShowEntries(greek, "Beta"); ShowEntries(greek, "Gamma"); } static void ShowEntries(BiDictionary<int, string> dict, string key) { IList<int> values = dict[key]; StringBuilder builder = new StringBuilder(); foreach (int value in values) { if (builder.Length != 0) { builder.Append(", "); } builder.Append(value); } Console.WriteLine("{0}: [{1}]", key, builder); }}
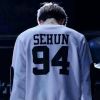
白衣非少年
TA贡献1155条经验 获得超0个赞
我刚刚注意到您想要从Value映射到多个键-我把这个解决方案留给单个值版本,但是接下来我将为多条目双向映射添加另一个答案。
using System;using System.Collections.Generic;class BiDictionary<TFirst, TSecond>{ IDictionary<TFirst, TSecond> firstToSecond = new Dictionary<TFirst, TSecond>(); IDictionary<TSecond, TFirst> secondToFirst = new Dictionary<TSecond, TFirst>(); public void Add(TFirst first, TSecond second) { if (firstToSecond.ContainsKey(first) || secondToFirst.ContainsKey(second)) { throw new ArgumentException("Duplicate first or second"); } firstToSecond.Add(first, second); secondToFirst.Add(second, first); } public bool TryGetByFirst(TFirst first, out TSecond second) { return firstToSecond.TryGetValue(first, out second); } public bool TryGetBySecond(TSecond second, out TFirst first) { return secondToFirst.TryGetValue(second, out first); }}class Test{ static void Main() { BiDictionary<int, string> greek = new BiDictionary<int, string>(); greek.Add(1, "Alpha"); greek.Add(2, "Beta"); int x; greek.TryGetBySecond("Beta", out x); Console.WriteLine(x); }}
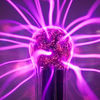
MM们
TA贡献1886条经验 获得超2个赞
var greek = new Dictionary<int, string> { { 1, "Alpha" }, { 2, "Alpha" } };
greek.WhatDoIPutHere("Alpha")
?
IEnumerable<T>
IEnumerable<TKey> KeysFromValue<TKey, TValue>(this Dictionary<TKey, TValue> dict, TValue val){ if (dict == null) { throw new ArgumentNullException("dict"); } return dict.Keys.Where(k => dict[k] == val);}var keys = greek.KeysFromValue("Beta"); int exceptionIfNotExactlyOne = greek.KeysFromValue("Beta").Single();
- 3 回答
- 0 关注
- 616 浏览
添加回答
举报
0/150
提交
取消