2 回答
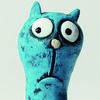
TA贡献1799条经验 获得超9个赞
您可以改用groupby:
from operator import itemgetter
from itertools import groupby
def most_common_name(L):
l = sorted(L)
it = map(lambda pair: (pair[0], len(list(pair[1]))), groupby(l))
r, _ = max(it, key=itemgetter(1))
return r
result = most_common_name(['dan', 'david', 'dan', 'jen', 'james'])
print(result)
输出
dan
或者更易读的替代方案:
from itertools import groupby
def len_group(pair):
return sum(1 for _ in pair[1])
def most_common_name(l):
sorted_l = sorted(l)
r, _ = max(groupby(sorted_l), key=len_group)
return r
result = most_common_name(['dan', 'david', 'dan', 'jen', 'james'])
print(result)
在这两种选择中,想法是 groupby 处理连续值的分组。然后你可以找到最大的组并返回该组的键。这些解决方案是O(nlogn),如果您对O(n)解决方案感兴趣,您可以使用 Counter 进行以下操作:
from operator import itemgetter
from collections import Counter
def most_common_name(l):
counts = Counter(l)
r, _ = max(counts.items(), key=itemgetter(1))
return r
result = most_common_name(['dan', 'david', 'dan', 'jen', 'james'])
print(result)
输出
dan
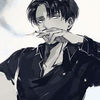
TA贡献1828条经验 获得超3个赞
稍微干净一点,同时保持相同的算法:
def mostCommonName(L):
if len(L) == 0:
return None
newL = sorted(L)
occurrences, current_name = 1, newL[0]
best_occurrences, best_name = 1, newL[0]
for i in range(1, len(newL)):
if newL[i] == current_name:
occurrences += 1
else:
if occurrences > best_occurrences:
best_occurrences, best_name = occurrences, current_name
occurrences = 1
current_name = newL[i]
return best_name
或者:
from collections import Counter
def mostCommonName(L):
return Counter(L).most_common(1)[0][0]
添加回答
举报