2 回答
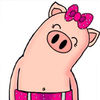
TA贡献2039条经验 获得超7个赞
可能有多种解决方案。一个简单的解决方案是使用指定的 goroutine 进行计算,您可以通过在通道上发送值来表示需要重新计算。发送可能是非阻塞的,因此,如果计算正在进行中,则不会发生任何事情。
下面是一个可重用的缓存实现:
type cache struct {
mu sync.RWMutex
value interface{}
updated time.Time
calcCh chan struct{}
expiration time.Duration
}
func NewCache(calc func() interface{}, expiration time.Duration) *cache {
c := &cache{
value: calc(),
updated: time.Now(),
calcCh: make(chan struct{}),
}
go func() {
for range c.calcCh {
v := calc()
c.mu.Lock()
c.value, c.updated = v, time.Now()
c.mu.Unlock()
}
}()
return c
}
func (c *cache) Get() (value interface{}, updated time.Time) {
c.mu.RLock()
value, updated = c.value, c.updated
c.mu.RUnlock()
if time.Since(updated) > c.expiration {
// Trigger a new calculation (will happen in another goroutine).
// Do non-blocking send, if a calculation is in progress,
// this will have no effect
select {
case c.calcCh <- struct{}{}:
default:
}
}
return
}
func (c *cache) Stop() {
close(c.calcCh)
}
注意:是停止背景戈鲁廷。呼叫后,不得调用。Cache.Stop()Cache.Stop()Cache.Get()
将其用于您的情况:
func getCachedScore() interface{} {
// ...
}
var scoreCache = NewCache(getCachedScore, 5*time.Second)
func serveScore(w http.ResponseWriter, r* http.Request) {
score, _ := scoreCache.Get()
b, _ := json.Marshal(score)
w.Write(b)
}
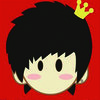
TA贡献1898条经验 获得超8个赞
这是我实现的,与icza的答案相关,但具有更多功能:
package common
import (
"context"
"sync/atomic"
"time"
)
type (
CachedUpdater func() interface{}
ChanStruct chan struct{}
)
type Cached struct {
value atomic.Value // holds the cached value's interface{}
updatedAt atomic.Value // holds time.Time, time when last update sequence was started at
updatePeriod time.Duration // controls minimal anount of time between updates
needUpdate ChanStruct
}
//cachedUpdater is a user-provided function with long expensive calculation, that gets current state
func MakeCached(ctx context.Context, updatePeriod time.Duration, cachedUpdater CachedUpdater) *Cached {
v := &Cached{
updatePeriod: updatePeriod,
needUpdate: make(ChanStruct),
}
//v.updatedAt.Store(time.Time{}) // "was never updated", but time should never be nil interface
v.doUpdate(time.Now(), cachedUpdater)
go v.updaterController(ctx, cachedUpdater)
return v
}
//client will get cached value immediately, and optionally may trigger an update, if value is outdated
func (v *Cached) Get() interface{} {
if v.IsExpired(time.Now()) {
v.RequestUpdate()
}
return v.value.Load()
}
//updateController goroutine can be terminated both by cancelling context, provided to maker, or by closing chan
func (v *Cached) Stop() {
close(v.needUpdate)
}
//returns true if value is outdated and updater function was likely not called yet
func (v *Cached) IsExpired(currentTime time.Time) bool {
updatedAt := v.updatedAt.Load().(time.Time)
return currentTime.Sub(updatedAt) > v.updatePeriod
}
//requests updaterController to perform update, using non-blocking send to unbuffered chan. controller can decide not to update in case if it has recently updated value
func (v *Cached) RequestUpdate() bool {
select {
case v.needUpdate <- struct{}{}:
return true
default:
return false
}
}
func (v *Cached) updaterController(ctx context.Context, cachedUpdater CachedUpdater) {
for {
select {
case <-ctx.Done():
return
case _, ok := <-v.needUpdate:
if !ok {
return
}
currentTime := time.Now()
if !v.IsExpired(currentTime) {
continue
}
v.doUpdate(currentTime, cachedUpdater)
}
}
}
func (v *Cached) doUpdate(currentTime time.Time, cachedUpdater CachedUpdater) {
v.updatedAt.Store(currentTime)
v.value.Store(cachedUpdater())
}
- 2 回答
- 0 关注
- 61 浏览
添加回答
举报