3 回答
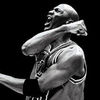
TA贡献1847条经验 获得超11个赞
我更喜欢两种将变量传递给子组件的方法。它们在不同的情况下都有用
方法一:使用属性 => Props
如果您的组件树嵌套不深,则此方法很有用。例如,您希望将变量从父项传递给子项。
一个嵌套的组件如下
const ParentComponent = () => {
const [variable, setVariable] = useState(0);
return (
<ChildComponent variable={variable} setVariable={setVariable} /> //nested within ParentComponent, first level
)
}
const ChildComponent = (props) => {
return(
<>
<div>prop value is {props.variable}</div> //variable attribute available through component props
<button onClick={props.setVariable(prevValue => prevValue+1}>add +1</button> //set value in parent through callBack method
</>
)
}
如果你有一个高度嵌套的组件层次结构,事情就会变得有点混乱。比方说, ChildComponent 返回另一个组件,并且您希望variable将 传递给该组件,但是 ChildComponent 不需要该变量,您最终会遇到这种情况
const ParentComponent = () => {
const [variable, setVariable] = useState(false);
return (
<ChildComponent someProp={variable}/> //nested within ParentComponent, first level
)
}
const ChildComponent = (props) => {
return(
<AnotherCustomComponent someProp={props.someProps}/> //someProp attribute available through component props
)
}
const AnotherCustomComponent = (props) => {
return(
<div>prop value is {props.someProp}</div> //someProp attribute available through component props
)
}
即使 ChildComponent 不需要该道具,它也需要通过道具将其推送到其子组件。这被称为“螺旋桨钻井”。这是一个简单的示例,但对于更复杂的系统,它可能会变得非常混乱。为此,我们使用...
方法二:Context API CodeSandbox
上下文 API 提供了一种向子组件提供状态的巧妙方法,而不会以道具钻井情况结束。它需要一个Provideris setup,它将它的值提供给它的任何Consumers'. Any component that is a child of the Provider 可以使用上下文。
首先创建一段上下文。
CustomContext.js
import React from 'react';
const CustomContext = React.createContext();
export function useCustomContext() {
return React.useContext(CustomContext);
}
export default CustomContext;
接下来是实现提供者,并给它一个值。我们可以使用之前的 ParentComponent 并添加 Context provider
import CustomContext from './CustomContext'
const ParentComponent = () => {
const [variable, setVariable] = useState(false);
const providerState = {
variable,
setVariable
}
return (
<CustomContext.Provider value={providerState} >
<ChildComponent />
</CustomContext.Provider>
)
}
现在任何嵌套在 <CustomContext.Provider></CustomContext.Provider> 中的组件都可以访问传递到Provider
我们嵌套的子组件看起来像这样
const ChildComponent = (props) => {
return(
<AnotherCustomComponent/> //Notice we arent passing the prop here anymore
)
}
const AnotherCustomComponent = (props) => {
const {variable, setVariable} = useCustomContext(); //This will get the value of the "value" prop we gave to the provider at the parent level
return(
<div>prop value is {variable}</div> //variable pulled from Context
)
}
如果 ParentComponent 被使用两次,则 ParentComponent 的每个实例都将有自己的“CustomContext”可供其子组件使用。
const App() {
return (
<>
<ParentComponent/>
<ParentComponent/>
</>
}
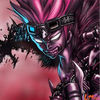
TA贡献1794条经验 获得超8个赞
您可以使用回调函数来执行此操作。
您基本上将一个函数传递给您的子组件,您的子组件将触发该函数,而父组件将具有该值。
这是一个应该如何实现的简单示例:
家长:
const Parent = () => {
const onSearchResult = searchResults => {
console.log(searchResults)
}
return (
<>
I am the parent component
<Child onSearchResult={onSearchResult} />
</>
)
}
孩子:
const Child = onSearchResult => {
const calculateResults = e => {
const results = doSomeStuff(e)
onSearchResult(results)
}
return (
<>
I am the child component
I have a component that will return some value
<input onKeyPress={e => calculateResults(e)}
</>
)
}
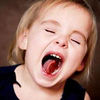
TA贡献1777条经验 获得超10个赞
子组件应该从父组件那里获取一个回调属性。有点像按钮的工作方式:
<Button onClick={this.onButtonClick}
你想要的是做
<SearchComponent onSearchResults={this.onResults}
然后,在搜索组件中,您可以调用this.props.onSearchResults(searchResults);
添加回答
举报