3 回答
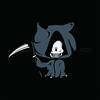
TA贡献1848条经验 获得超10个赞
这是引入自定义Undefined类(作为单例)的另一种方法。此外,请确保键入您的类属性:
class Undefined
{
private static Undefined $instance;
protected function __constructor()
{
}
protected function __clone()
{
}
public function __wakeup()
{
throw new Exception("Not allowed for a singleton.");
}
static function getInstance(): Undefined
{
return self::$instance ?? (self::$instance = new static());
}
}
class Person
{
private int $age;
public function getAge(): int|Undefined
{
return $this->age ?? Undefined::getInstance();
}
}
$person = new Person();
if ($person->getAge() instanceof Undefined) {
// do something
}
但使用单例模式有一个缺点,因为应用程序中所有未定义的对象将严格彼此相等。否则,每个返回未定义值的get 操作都会产生副作用,即另一块分配的 RAM。
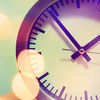
TA贡献1848条经验 获得超2个赞
PHP 不像 javascript 那样具有未定义的值。但它不是严格类型的,所以如果您没有找到更好的解决方案,这里有一个自定义类型 UNDEFINED
<?php
class UNDEFINED { }
class Test {
var $a;
function __construct( $a='' ) {
$this->a = new UNDEFINED();
if( $a !== '' ) {
$this->a = $a;
}
}
function isDefined() {
$result =true;
if(gettype($this->a) === 'object'){
if(get_class($this->a) === 'UNDEFINED') {
$result=false;
}
}
echo gettype($this->a) . get_class($this->a);
return $result;
}
}
$test= new Test();
$test->isDefined();
这是一个可能更好的版本,它使用 instanceof 而不是 get_call 和 getType
<?php
class UNDEFINED { }
class Test {
var $id;
var $a;
var $b;
function __construct( $id) {
$this->id = $id;
$this->a = new UNDEFINED();
$this->b = new UNDEFINED();
}
function init( $a = '' , $b = '') {
$this->a = $this->setValue($a,$this->a);
$this->b = $this->setValue($b,$this->b);
}
function setValue($a,$default) {
return $a === '' ? $default : $a;
}
function isUndefined($a) {
return $a instanceof UNDEFINED;
}
public function isFullyLoaded()
{
$result = true;
$properties = get_object_vars($this);
print_r($properties);
foreach ($properties as $property){
$result = $result && !$this->isUndefined($property);
if ( !$result) break;
}
return $result;
}
function printStatus() {
if($this->isFullyLoaded() ) {
echo 'Loaded!';
} else {
echo 'Not loaded';
}
}
}
$test= new Test(1);
$test->printStatus();
$test->init('hello');
$test->printStatus();
$test->init('', null);
$test->printStatus();
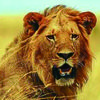
TA贡献1828条经验 获得超4个赞
用途property_exists():
<?php
error_reporting(E_ALL);
// oop:
class A {
public $null_var = null;
}
$a = new A;
if(property_exists($a, 'null_var')) {
echo "null_var property exists\n";
}
if(property_exists($a, 'unset_var')) {
echo "unset_var property exists\n";
}
// procedural:
$null_var = null;
if(array_key_exists('null_var', $GLOBALS)) {
echo "null_var variable exists\n";
}
if(array_key_exists('unset_var', $GLOBALS)) {
echo "unset_var variable exists\n";
}
// output:
// null_var property exists
// null_var variable exists
- 3 回答
- 0 关注
- 66 浏览
添加回答
举报