3 回答
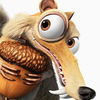
TA贡献1829条经验 获得超13个赞
ButtonHandler1并没有真正使用,因为传递给它的参数从未使用过,并且在构造它时它的单个方法被覆盖。
所以这:
butSmall.addActionListener(new ButtonHandler1(this, 200){
@Override
public void actionPerformed(ActionEvent actionEvent) {
size = 200;
panel.repaint();
}
});
无需构建即可编写ButtonHandler1:
butSmall.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent actionEvent) {
size = 200;
panel.repaint();
}
});
或者使用 lambda 表达式:
butSmall.addActionListener(actionEvent -> {
size = 200;
panel.repaint();
});
有很多方法可以实现您想要的功能。根据您所写的内容,您可以ButtonHandler1这样定义:
class ButtonHandler1 implements ActionListener{
private final FilledFrame theApp;
private final int theSize;
ButtonHandler1(FilledFrame app, int size){
theApp = app;
theSize = size;
}
@Override
public void actionPerformed(ActionEvent actionEvent) {
theApp.size = theSize; //better use a setter in FilledFrame
theApp.repaint();
}
}
并像这样使用它:
butSmall.addActionListener(new ButtonHandler1(this, 200));
butMedium.addActionListener(new ButtonHandler1(this, 300));
butLarge.addActionListener(new ButtonHandler1(this, 400));
创建ButtonHandler1一个内部类FilledFrame使事情变得更简单:
class ButtonHandler1 implements ActionListener{
private final int theSize;
ButtonHandler1(int size){
theSize = size;
}
@Override
public void actionPerformed(ActionEvent actionEvent) {
size = theSize;
repaint();
}
}
通过以下方式使用它:
butSmall.addActionListener(new ButtonHandler1(200));
butMedium.addActionListener(new ButtonHandler1(300));
butLarge.addActionListener(new ButtonHandler1(400));
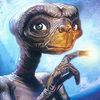
TA贡献1802条经验 获得超5个赞
a 的任何处理程序*Listener都用于处理events从正在侦听的对象生成的处理程序。在主程序中,似乎有几个可以改变窗口大小的按钮。
对于实现 ButtonHandler 的类,您没有执行任何操作,因为您的actionPerformed方法没有执行任何操作。
并且anonymous classes是实现侦听器的可接受的方式。但是,我更喜欢使用,inner classes因为它们更干净(恕我直言)并且仍然可以state访问enclosing class.
您的处理程序类应如下所示:
// This is a class whose object will handle the event.
class ButtonHandler1 implements ActionListener {
private FilledFrame theApp;
private int theSize;
ButtonHandler1(FilledFrame app, int size) {
theApp = app;
theSize = size;
}
public void actionPerformed(ActionEvent actionEvent) {
theApp.size = theSize;
theApp.repaint();
}
}
以下是将该处理程序添加到按钮的调用。
butSmall.addActionListener(new ButtonHandler1(this, 200));
butMedium.addActionListener(new ButtonHandler1(this, 300));
butLarge.addActionListener(new ButtonHandler1(this, 400));
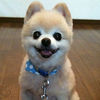
TA贡献1806条经验 获得超8个赞
我不确定我是否理解这个问题,但有两种方法可以重构代码:
完全删除您的ButtonHandler1类并实现匿名类中的所有逻辑:
//FilledFrame.java
butSmall.addActionListener(new ActionListener(){
@Override
public void actionPerformed(ActionEvent actionEvent) {
FilledFrame.this.size = 200;
panel.repaint();
}
});
您可以在类上添加一些 getter 和 setter 方法FilledFrame,并调用它们ButtonHandler1.actionPerformed来实现其中的逻辑,如下所示:
package Lab2;
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
// This is a class whose object will handle the event.
public class ButtonHandler1 implements ActionListener{
private FilledFrame theApp;
private int theSize;
ButtonHandler1(FilledFrame app, int size){
theApp = app;
theSize = size;
}
public void actionPerformed(ActionEvent actionEvent) {
theApp.setSizeMember(200); //do not use theApp.setSize(), create a method with a different name
theApp.getSquarePanel().repaint();
}
}
我将创建 getter/setter 的工作留给FilledFrame您。
添加回答
举报