5 回答
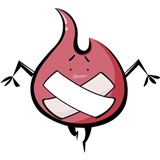
TA贡献1836条经验 获得超5个赞
使用嵌套循环。
nums = [8,1,2,2,3];
answer = [];
for (let i = 0; i < nums.length; i++) {
let count = 0;
for (let j = 0; j < nums.length; j++) {
if (nums[j] < nums[i]) {
count++;
}
}
answer.push(count);
console.log(`For nums[${i}]=${nums[i]} there are ${count} lower numbers`);
}
console.log(`Answer: ${answer}`);
没有必要进行测试i != j
,因为数字永远不会低于自身。
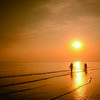
TA贡献1998条经验 获得超6个赞
一种更简单的方法是简单地对数组进行排序,然后元素的索引会告诉你有多少比它少:
const nums = [8,1,2,2,3]
const sorted = [...nums].sort();
const result = nums.map((i) => {
return sorted.findIndex(s => s === i);
});
console.log(result);
这样做的另一个好处是您不必为每个数字搜索整个数组。
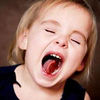
TA贡献1777条经验 获得超10个赞
一种方法是在值小于当前值的条件下过滤数组,然后统计过滤后的数组中值的个数:
const nums = [8,1,2,2,3];
const smallerNums = nums.map(v => nums.filter(n => n < v).length);
console.log(smallerNums); // [4,0,1,1,3]
或者,您可以在 reduce 中进行计数,这应该会快得多:
const nums = [8, 1, 2, 2, 3];
const smallerNums = nums.map(v => nums.reduce((c, n) => c += (n < v), 0));
console.log(smallerNums); // [4,0,1,1,3]
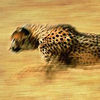
TA贡献1851条经验 获得超3个赞
我喜欢:
function rankZero(array){
const s = [...array], r = [];
s.sort((a, b)=>{
return a - b;
});
for(let n of array){
r.push(s.indexOf(n));
}
return r;
}
console.log(rankZero([8, 1, 2, 2, 3]));
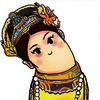
TA贡献1842条经验 获得超12个赞
我对每个解决方案进行了性能测试。在我的电脑上(Intel Core I9-9900,64GB RAM)@StackSlave 的解决方案一直是最快的,其次是其他排序解决方案、reduce 解决方案、基本迭代和过滤器。您可以在下面自己运行测试:
const datalength = 1000;
const iterations = 100;
const getRandom = (min, max) => Math.random() * (max - min) + min;
const data = Array.from({
length: datalength
}, () => getRandom(1, 100));
const mapper = arr => arr.map(i => arr.filter(n => n < i).length);
const sorter = nums => {
const sorted = [...nums].sort();
const result = nums.map((i) => {
return sorted.findIndex(s => s === i);
});
};
const iterator = arr => {
const answer = [];
for (let i = 0; i < arr.length; i++) {
let count = 0;
for (let j = 0; j < arr.length; j++) {
if (arr[j] < arr[i]) {
count++;
}
}
answer.push(count);
}
return answer;
};
const rankZero = array => {
const s = [...array],
r = [];
s.sort((a, b) => {
return a - b;
});
for (let n of array) {
r.push(s.indexOf(n));
}
return r;
}
const reducer = arr => arr.map(v => arr.reduce((c, n) => c += (n < v), 0));
let fns = {
'iterator': iterator,
'mapper': mapper,
'sorter': sorter,
'reducer': reducer,
'rankZero': rankZero
}
for (let [name, fn] of Object.entries(fns)) {
let total = 0;
for (i = 0; i < iterations; i++) {
let t0 = performance.now();
fn(data);
let t1 = performance.now();
total += t1 - t0;
}
console.log(name, (total / iterations).toFixed(2));
}
添加回答
举报